Today We will complete one end-to-end dotnet 7 project. In this dotnet 7 project, we will cover the
following topics mentioned below.
Table of Contents
ToggleWhat’s New is Dotnet 7?
.NET 7 is the successor to .NET 6 and focuses
on being unified, modern, simple, and fast. .NET 7 will be supported for 18 months as a
standard-term support (STS) release (previously known as a current release). Performance is a key focus of .NET 7, and all of its features are designed with performance in mind.
You can read about the features of .Net 7 from here
Now Let’s jump to our content. I would strongly recommend you follow each and every line below so you can create one bug-free dotnet 7 project.
What you’ll learn in .Net 7 Project
- Learn, Understand, and Create ASPNET Core Web API From Scratch using .NET 7
- Building scalable REST APIs from scratch using ASPNET CORE and C#
- Learn and Apply Entity Framework Core to perform CRUD operations on a SQL Server database
- Use Entity Framework Core in a code-first approach
- Understand and Apply the Repository Pattern in ASPNET Core Web API
- Use Domain Driven Design (DDD) approach to create domain-first models and project
- Understand RESTful Principles and Apply them in ASPNET Core Web API
- Understand Best practices and Clean Coding Techniques, Know Shortcuts and Tips and Tricks
- Add Validations In ASPNET CORE Web API
- Use popular third-party libraries such as AUTOMAPPER
- Understand and Use Interfaces, Inheritance, Dependency Injection etc
- Understand and Implement Authentication and Role-based Authorization to Authenticate and Authorize the ASPNET Core Database
- Create JWT tokens to Authenticate API
- Test ASPNET Core Web API using Swagger and Postman
- Use ASPNET Core Identity in ASPNET Core Web API to Authenticate and add Role-based Authorizatio
Requirements to complete dotnet 7 project
- Hello world experience in ASPNET Core
- Beginner knowledge about APIs and their use
- 3-6 months experience working with ASPNET Core and C#
Create a New dotnet 7 project
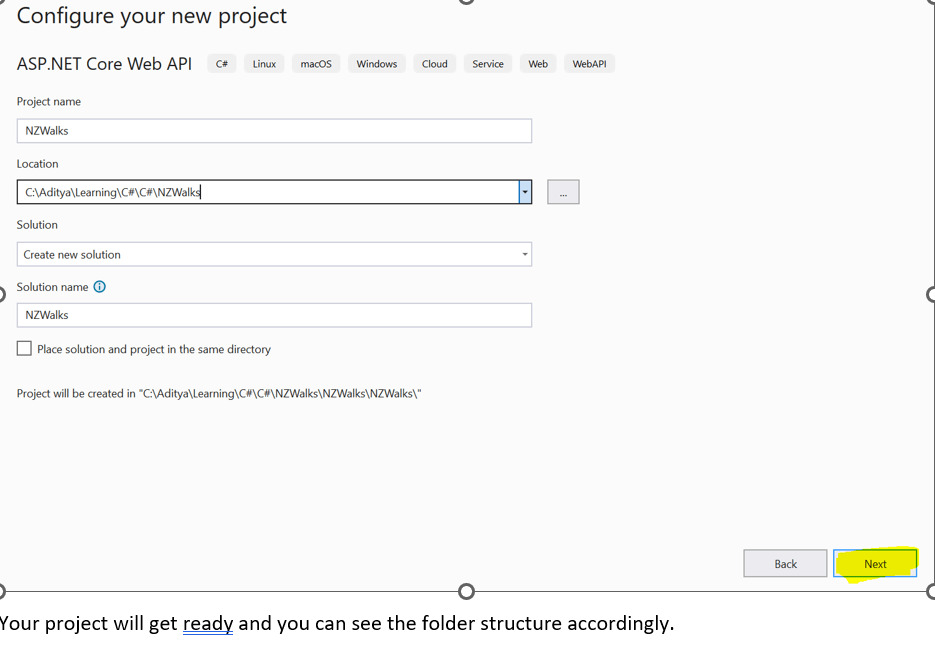
Basic Folder Structure
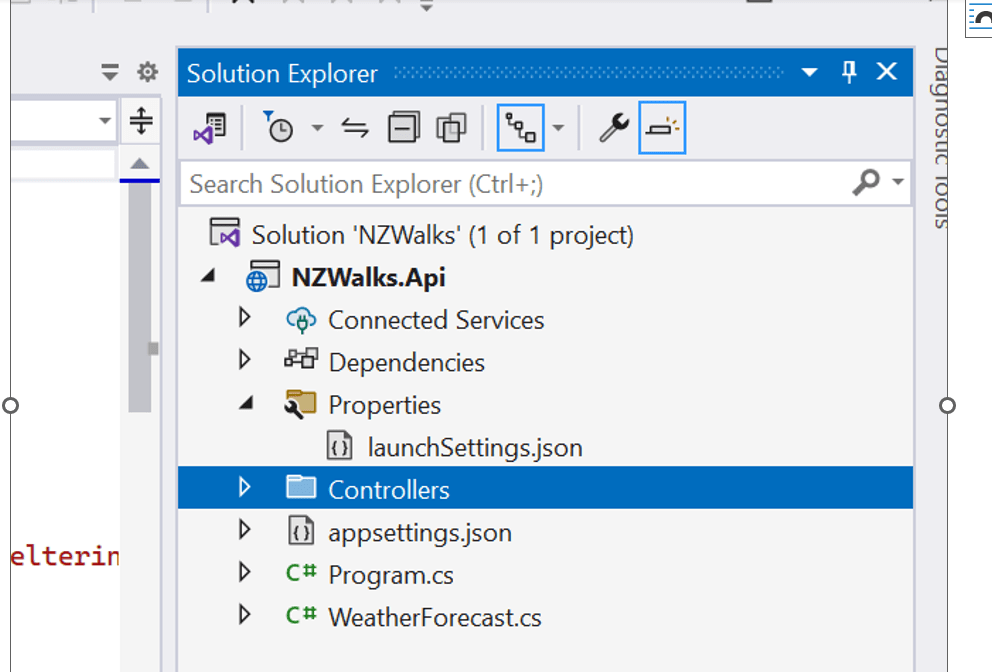
Click on RUN button and your dotnet 7 project will launch its UI like below
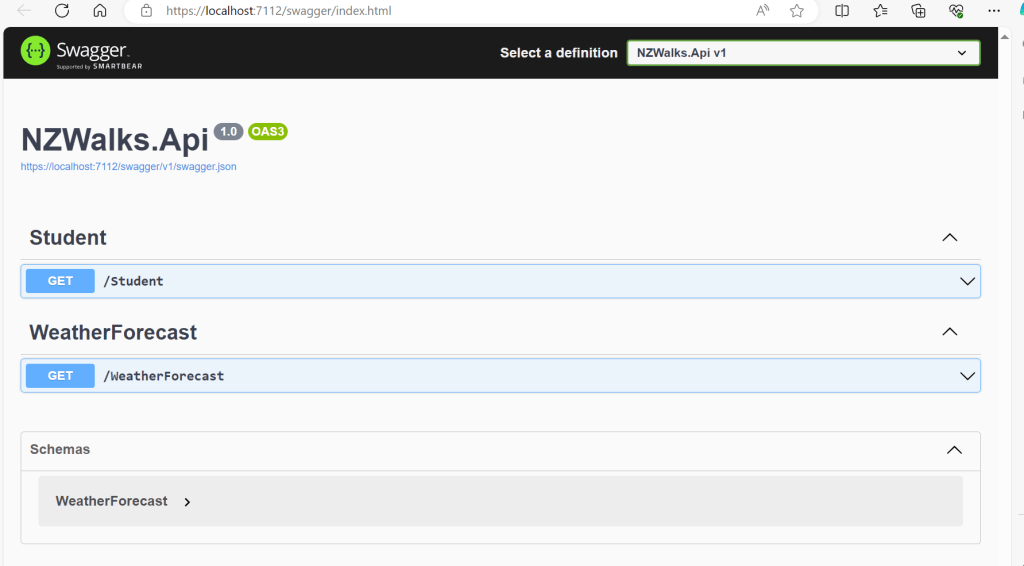
You can run your application from run button, and you will see the output page like this. This is SWAGGER page where you can hit your API if it is running fine or not.
Note: I have created one more API with /Student controller, this is the reason you are seeing 2 API. In your case it might be only one..
If you also want to create the sample API, then you can follow these few steps:
Right Click on Controller à Click on Add New à Name your controller as StudentController à Create
Update the StudentController with the below code, now you will also able to see /Student API in Swagger.
StudentController.cs
using Microsoft.AspNetCore.Mvc;
namespace NZWalks.Api.Controllers
{
[Route("/[controller]")]
[ApiController]
public class StudentController : Controller
{
[HttpGet]
public IActionResult GetAllStudent()
{
string[] student = new string[] { "john", "Aditya" };
return Ok(student);
}
}
}
Now, let’s create the Domain class, for I will create a new folder Model, and then inside that I will create a Domain folder, and inside the domain folder, will create the Difficulty.cs, Region.cs, Walk.cs class. Below is the folder structure screenshot for the same.
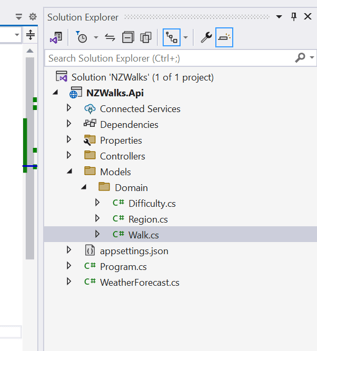
Difficulty.cs
namespace NZWalks.Api.Models.Domain
{
public class Difficulty
{
public Guid Id { get; set; }
public string Name { get; set; }
}
}
Region.cs
namespace NZWalks.Api.Models.Domain
{
public class Region
{
public Guid Id { get; set; }
public string Code { get; set; }
public string Name { get; set; }
public string? RegionImageUrl { get; set; }
}
}
Walk.cs
namespace NZWalks.Api.Models.Domain
{
public class Walk
{
public Guid Id { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public double LengthInKm { get; set; }
public string? WalkImageUrl { get; set; }
public Guid DifficultyId { get; set; }
public Difficulty Difficulty { get; set; }
public Region Region { get; set; }
}
}
Now, we will install 2 package to run our dotnet 7 Api
project.
Go to Dependency -> Nuget Package à install below 2 package
- Microsoft.EntityFrameworkCore.SQlServer
- Microsoft.EntityFrameworkCore.Tools
Now lets create the DbContext Class (part of Entity core package):
It will be used for performing CRUD operations basically. It is the primary
class to interact with database.
Create a folder with Data in our own dotnet 7 project, inside that folder create a class with name NzWalkDbContext.cs and paste the below code
NzWalkDbContext.cs
using Microsoft.EntityFrameworkCore;
using NZWalks.Api.Models.Domain;
namespace NZWalks.Api.Data
{
public class NzWalksDbContext : DbContext
{
public NzWalksDbContext(DbContextOptions dbContextOptions) : base(dbContextOptions)
{
}
public DbSet Difficulties { get; set; }
public DbSet Regions { get; set; }
public DbSet Walks { get; set; }
}
}
Now, we will add the Db Connection in appsetting.json file. In pace of my server name, add your server name(you will find this in SQL Server )
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"NzWalksConnectionString": "Server=SHerfdf1;Database=NZWalksdB;Trusted_Connection=true;TrustServerCertificate=True"
}
}
Now we will use dependency injection and inject our dB class into the program.cs. Program class is the entry point of any dotnet application.
Program.cs
using Microsoft.EntityFrameworkCore;
using NZWalks.Api.Data;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
builder.Services.AddDbContext(options =>
options.UseSqlServer(builder.Configuration.GetConnectionString("NzWalksConnectionString")));
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
Now, migrate the db. To migrate the db we will use the below command one by one in the package manager console (View à Other Window à Package Manager Console)
- Add-Migration “Initial Migration”
- Update-Database
After successful of this command, you will find table has been created in dB and a new folder ‘migration’ has been created in the solution.
Now we will create few DTO Class and will call the API via DTO, updated dotnet 7 project folder looks like
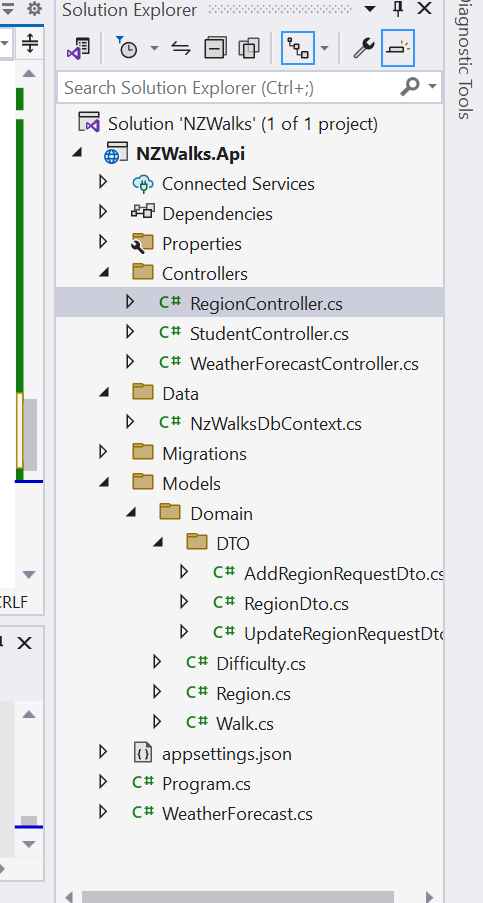
RegionDto.cs
namespace NZWalks.Api.Models.Domain.DTO
{
public class RegionDto
{
public Guid Id { get; set; }
public string Code { get; set; }
public string Name { get; set; }
public string? RegionImageUrl { get; set; }
}
}
AddRegionRequestDto.cs
namespace NZWalks.Api.Models.Domain.DTO
{
public class AddRegionRequestDto
{
public string Code { get; set; }
public string Name { get; set; }
public string? RegionImageUrl { get; set; }
}
}
UpdateRegionRequestDto.cs
namespace NZWalks.Api.Models.Domain.DTO
{
public class UpdateRegionRequestDto
{
public string Code { get; set; }
public string Name { get; set; }
public string? RegionImageUrl { get; set; }
}
}
We have also created the Controller class for Region, Difficulty, and Walk.cs class and will perform CRUD operation on all 3 controllers. Create the folder and controller same as above screenshot.
To Create the Controller, Right click on Controller — Add New Controller– Select API — Choose API Controller- Empty.
In the below code snippet ‘RegionController.cs’, we have completed CRUD for our dotnet 7 project. We have use all the verb i.e GET, POST, PUT, DELETE. If you want to learn CRUD in dotnet 7 project , you can follow below.
RegionController.cs
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using NZWalks.Api.Data;
using NZWalks.Api.Models.Domain;
using NZWalks.Api.Models.Domain.DTO;
namespace NZWalks.Api.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class RegionController : ControllerBase
{
private readonly NzWalksDbContext dbContext;
public RegionController(NzWalksDbContext dbContext)
{
this.dbContext = dbContext;
}
//Get All Region from dB
[HttpGet]
public IActionResult GetAll()
{
//Get Details from dB
var regionsDomain = dbContext.Regions.ToList();
//Map Domain model to DTO
var regionsDto = new List();
foreach (var regionDomain in regionsDomain)
{
regionsDto.Add(new RegionDto()
{
Id = regionDomain.Id,
Code = regionDomain.Code,
Name = regionDomain.Name,
RegionImageUrl = regionDomain.RegionImageUrl,
});
}
return Ok(regionsDto);
}
//Get All Region from dB by ID
//GET: https://localhost:port/api/regions/{id]
[HttpGet]
[Route("{id:Guid}")]
public IActionResult GetRegionById([FromRoute] Guid id)
{
//Getting from dB
var regionDomain = dbContext.Regions.Find(id);
if (regionDomain == null)
{
return NotFound();
}
//Map to RegionDto
var regionDto = new RegionDto
{
Id = regionDomain.Id,
Code = regionDomain.Code,
Name = regionDomain.Name,
RegionImageUrl = regionDomain.RegionImageUrl,
};
//Return DTO back to client
return Ok(regionDto);
}
//Post to create new Region
[HttpPost]
public IActionResult Create([FromBody] AddRegionRequestDto addRegionRequestDto)
{
//Map Dto to Domain Model
var regionDomainModel = new Region
{
Code = addRegionRequestDto.Code,
Name = addRegionRequestDto.Name,
RegionImageUrl = addRegionRequestDto.RegionImageUrl,
};
//Use Domain model to create Region
dbContext.Regions.Add(regionDomainModel);
dbContext.SaveChanges();
//Map Domain model back to Dto
var regionsDto = new RegionDto
{
Id = regionDomainModel.Id,
Code = regionDomainModel.Code,
Name = regionDomainModel.Name,
RegionImageUrl = regionDomainModel.RegionImageUrl,
};
return CreatedAtAction(nameof(GetRegionById), new { id = regionsDto.Id }, regionsDto);
}
//Update Region
//PUT
[HttpPut]
[Route("{id:Guid}")]
public IActionResult Update([FromRoute] Guid id, [FromBody] UpdateRegionRequestDto updateRegionRequestDto)
{
//Check if region exist
var regionDomainModel= dbContext.Regions.FirstOrDefault(x => x.Id == id);
if(regionDomainModel == null)
{
return NotFound();
}
//Map Dto to Domain Model
regionDomainModel.Code = updateRegionRequestDto.Code;
regionDomainModel.Name = updateRegionRequestDto.Name;
regionDomainModel.RegionImageUrl = updateRegionRequestDto.RegionImageUrl;
dbContext.SaveChanges();
//Convet Domain model to dto
var regionDto = new RegionDto
{
Id = regionDomainModel.Id,
Code = regionDomainModel.Code,
Name = regionDomainModel.Name,
RegionImageUrl = regionDomainModel.RegionImageUrl,
};
return Ok(regionDto);
}
[HttpDelete]
[Route("{id:Guid}")]
public IActionResult Delete([FromRoute] Guid id)
{
var regionDomainModel = dbContext.Regions.FirstOrDefault(x => x.Id == id);
if(regionDomainModel == null)
{
return NotFound();
}
dbContext.Regions.Remove(regionDomainModel);
dbContext.SaveChanges();
//return deleted region
return Ok();
}
}
}
If you are still here and completing this dotnet 7 project , i wanted to thank you for finding the post helpful. Also, if you want to have some finance and credit card idea, you can go through the below link
Next Part of the dotnet7 project
Dont Worry! Project not ended yet. Next part of the dotnet 7 project is here